Simple Array CRUD Manipulation Using AngularJS Framework
This weekend I decided to create a simple AngularJS CRUD array application. This example was based on Dan Wahlin's AngularJS tutorial. I simply reused the css and js files for simplicity sake. So, let's proceed with the actual scripts and html.
Main HTML (Contains the input controls and div with ng-view directive which is the container of the partial view:
Partial View: (Show objects in a table control)
Define Angular Module:
Config Script to handle routes:
Factory script that declares factory object, customer objects and index for the customer in edit mode:
Controller which defines the events such as adding a customer, deleting a customer and editing a customer:
Default UI:
Edit Mode:
Delete Item:
Add Customer:
Cheers!
Main HTML (Contains the input controls and div with ng-view directive which is the container of the partial view:
<div data-ng-controller="CustomerController"> <h3>CRUD application in AngularJS Array</h3> <hr /> Name:<br /> <input id="inName" type="text" data-ng-model="inputData.name" placeholder="name" /> <br /> City:<br /> <input id="inCity" type="text" data-ng-model="inputData.city" placeholder="city" /> <br /> <button class="btn btn-primary" data-ng-click="saveCustomer()">Save</button> <button class="btn btn-primary" data-ng-click="cancelCustomer()">Cancel</button> <br /> <br /> Filter by Name: <input id="inSearch" type="text" data-ng-model="nameText" /> <br /> <div data-ng-view=""></div> <hr /> </div>
<table class="tableData"> <tr> <th>Name</th> <th>Address</th> <th>Delete</th> <th>Edit</th> </tr> <tr data-ng-repeat="customer in customers | filter:nameText | orderBy:'name'"> <td> {{ customer.name }} </td> <td> {{ customer.city }} </td> <td> <button class="btn btn-primary" data-ng-click="removeCustomer(customer)">Delete</button> </td> <td> <button class="btn btn-primary" data-ng-click="editCustomer(customer)">Edit</button> </td> </tr> </table>
//'ngRoute' for routing var crudApp = angular.module('crudApp', ['ngRoute']);
//handle routes here.. crudApp.config(function ($routeProvider) { $routeProvider .when('/partial1', { templateUrl: '/AkoDemoPartials/8crudPartial.html', controller: 'CustomerController' }) .otherwise({ redirectTo: '/partial1' }); });
crudApp.factory('simpleFactory', function () { //create factory object var factory = {}; var itemIndex = -1; //index for handling edit var customers = [ { name: 'Dave Jones', city: 'Phoenix' }, { name: 'Jamie Riley', city: 'Atlanta' }, { name: 'Heedy Wahlin', city: 'Chandler' }, { name: 'Thomas Winter', city: 'Seattle' } ]; //getCustomers is simply going to come in and just return customers. factory.getCustomers = function () { //Can use $http object to retrieve remote data //in a "real" app return customers; }; factory.getSelectedIndex = function () { return itemIndex; } return factory; });
//pass the factory name crudApp.controller('CustomerController', function ($scope, simpleFactory) { $scope.customers = []; //perform initialization init(); function init() { $scope.customers = simpleFactory.getCustomers(); } $scope.saveCustomer = function () { //alert(simpleFactory.itemIndex); if (simpleFactory.itemIndex == -1 || simpleFactory.itemIndex == undefined) { if ($scope.inputData.name != "" && $scope.inputData.name != undefined && $scope.inputData.city != "" && $scope.inputData.name != undefined) { $scope.customers.push( { name: $scope.inputData.name, city: $scope.inputData.city }); } $scope.clearControls(); } else { //perform update on object //alert(simpleFactory.itemIndex + ',' + $scope.customers[simpleFactory.itemIndex].name + ',' + $scope.customers[simpleFactory.itemIndex].city); var customer = $scope.customers[simpleFactory.itemIndex]; customer.name = $scope.inputData.name; customer.city = $scope.inputData.city; $scope.clearControls(); simpleFactory.itemIndex = -1; } } $scope.removeCustomer = function (item) { var retVal = confirm("Do you want to delete item?"); if (retVal == true) { var index = $scope.customers.indexOf(item) $scope.customers.splice(index, 1); angular.element(document.querySelector('#inSearch')).val(""); angular.element(document.querySelector('#inSearch')).change(); if (index == simpleFactory.itemIndex) { simpleFactory.itemIndex = -1; } } } $scope.cancelCustomer = function () { $scope.clearControls(); simpleFactory.itemIndex = -1; } $scope.clearControls = function () { angular.element(document.querySelector('#inName')).val(""); angular.element(document.querySelector('#inCity')).val(""); $scope.triggerChangeEventInputControls(); } $scope.triggerChangeEventInputControls = function () { angular.element(document.querySelector('#inName')).change(); angular.element(document.querySelector('#inCity')).change(); } $scope.editCustomer = function (item) { simpleFactory.itemIndex = $scope.customers.indexOf(item); angular.element(document.querySelector('#inName')).val($scope.customers[simpleFactory.itemIndex].name); angular.element(document.querySelector('#inCity')).val($scope.customers[simpleFactory.itemIndex].city); $scope.triggerChangeEventInputControls(); } });

Edit Mode:
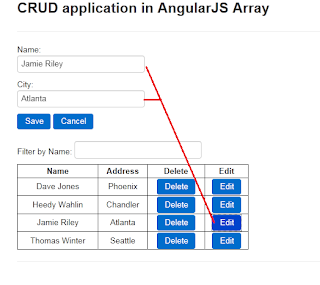
Delete Item:

Add Customer:


Cheers!
Is there a download anywhere for this tutorial?
ReplyDeleteGood tutorial !
Hi,
ReplyDeleteI based this on Dan Wahlin's AngularJS Fundamentals tutorial.
:)
Thanks for replying. Do you by any chance have the link to Dan's Tutorial? I have searched and can not find the one that matches this.
DeleteI wanted to look at your folder structure
Good job
Here's the link of the PDF tutorial: http://fastandfluid.com/publicdownloads/AngularJSIn60MinutesIsh_DanWahlin_May2013.pdf
ReplyDelete:)