Change WPF DataGridRow Background Color Using IValueConverter
There are several ways in painting a wpf datagrid. One option would be to use the IValueConverter interface. Firstly, you have to define a class that implements the interface. And add contracts to Convert() and ConvertBack() methods. Assuming in your form load, you bind a List object to the datagrid's ItemSource property. T could be a pre-defined class.
Here's the Resource code:
Here's the XAML markup:
Here's the Converter Class:
Here's a running datagrid window:
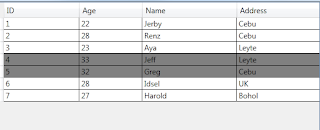
Cheers!
Here's the Resource code:
<Window.Resources>
<src:AgeTargetConverter x:Key="AgeTargetConverter" />
</Window.Resources>
<DataGrid Grid.Row="0" Grid.Column="0" AutoGenerateColumns="False" CanUserAddRows="False" Name="dgStudents"> <DataGrid.RowStyle> <Style TargetType="{x:Type DataGridRow}"> <Style.Triggers> <DataTrigger Binding="{Binding Age, Converter={StaticResource AgeTargetConverter}, ConverterParameter = 30}" Value="True"> <Setter Property="Background" Value="Gray"></Setter> </DataTrigger> </Style.Triggers> </Style> </DataGrid.RowStyle> <DataGrid.Columns> <DataGridTextColumn Header="ID" Binding="{Binding Path=ID}" Width="120" IsReadOnly="True" /> <DataGridTextColumn Header="Age" Binding="{Binding Path=Age}" MinWidth="100" IsReadOnly="True" /> <DataGridTextColumn Header="Name" Binding="{Binding Path=Name}" MinWidth="150" IsReadOnly="True" /> <DataGridTextColumn Header="Address" Binding="{Binding Path=Address}" MinWidth="150" IsReadOnly="True" /> </DataGrid.Columns> </DataGrid>
public class AgeTargetConverter : IValueConverter { public object Convert(object value, Type targetType, object parameter, System.GlobalizationCultureInfo culture) { int age = System.Convert.ToInt32(value); int maxAge = System.Convert.ToInt32(parameter); return (age >= maxAge); } public object ConvertBack(object value, Type targetType, object parameter, System.GlobalizationCultureInfo culture) { throw new NotImplementedException(); } }
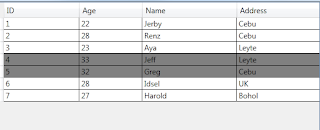
Cheers!
Comments
Post a Comment