DataGridView ComboBox Cascade In Windows Forms
Good day to all!
Here's an example of how to perform a combobox lookup or cascade using two DataGridViewComboBoxColumns inside a DataGridView control Combo Lookup in DGV. The solution presented is in VB.NET, so I decided to create a C# equivalent for this. In form load event, populate two DataTables for Roles and Employees. Each employee is assigned to a specific role.
The cascade code is performed in CellValueChanged event of the DataGridView.
Role Selection
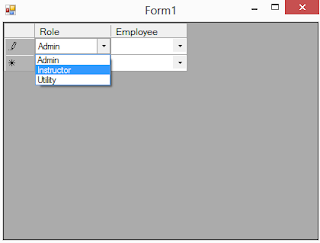
Employees Under Instructor Role
Here's an example of how to perform a combobox lookup or cascade using two DataGridViewComboBoxColumns inside a DataGridView control Combo Lookup in DGV. The solution presented is in VB.NET, so I decided to create a C# equivalent for this. In form load event, populate two DataTables for Roles and Employees. Each employee is assigned to a specific role.
private void Form1_Load(object sender, EventArgs e) { DataGridView1.Rows.Add(); dtRole.Columns.Add("RoleID"); dtRole.Columns.Add("RoleName"); dtRole.Rows.Add(1, "Admin"); dtRole.Rows.Add(2, "Instructor"); dtRole.Rows.Add(3, "Utility"); dtEmployee.Columns.Add("RoleID"); dtEmployee.Columns.Add("EmployeeID"); dtEmployee.Columns.Add("EmployeeName"); dtEmployee.Rows.Add(1, 1, "Sam"); dtEmployee.Rows.Add(1, 2, "Nicole"); dtEmployee.Rows.Add(2, 3, "Donald"); dtEmployee.Rows.Add(2, 4, "Brenda"); dtEmployee.Rows.Add(3, 5, "Jenny"); dtEmployee.Rows.Add(3, 6, "Michael"); Role.ValueMember = dtRole.Columns[0].ColumnName; Role.ValueType = typeof(string); Role.DisplayMember = dtRole.Columns[1].ColumnName; Role.DataSource = dtRole; }
private void DataGridView1_CellValueChanged(object sender, DataGridViewCellEventArgs e) { if (e.RowIndex >= 0 && ((DataGridView)sender).Columns[e.ColumnIndex].GetType() == typeof(DataGridViewComboBoxColumn)) { if (e.ColumnIndex == ((DataGridView)sender).Columns["Role"].Index) { if (((DataGridView)sender).Rows[e.RowIndex].Cells["Role"].Value != null) { DataView employeesDV = new DataView(dtEmployee); employeesDV.RowFilter = "RoleID = " + ((DataGridViewComboBoxCell)DataGridView1.Rows[e.RowIndex].Cells["Role"]).Value; //DataGridView1.CurrentCell.Value; employeesDV.RowStateFilter = DataViewRowState.CurrentRows; if (employeesDV.Count > 0) { DataGridViewComboBoxCell cell = (DataGridViewComboBoxCell)DataGridView1.Rows[e.RowIndex].Cells["Employee"]; cell.ValueMember = "EmployeeID"; Role.ValueType = typeof(int); cell.DisplayMember = "EmployeeName"; cell.DataSource = employeesDV; } } } } }
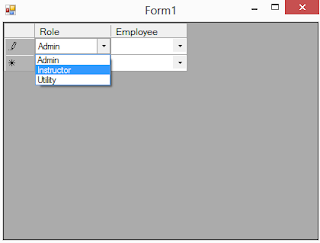
Employees Under Instructor Role

Comments
Post a Comment